Linux - AWK Command
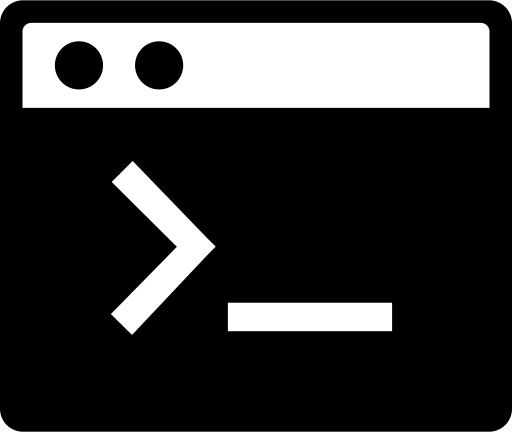
AWK is a line-oriented language basically used to manipulating data and generate reports. awk script no required any compiler to compile. In awk we use variables, numeric functions, string function and logical operators.
Awk is abbreviated from the names of the developers – Aho, Weinberger, and Kernighan.
An awk program operates on each line of an input file. Basic components are
- BEGIN { …. initialization awk commands …}
- { …. awk commands for each line of the file…}
- END { …. finalization awk commands …}
1ls -l | awk 'BEGIN {sum=0} {sum=sum+$5} END {print sum}'
Awk Variables
Variables | Description |
---|---|
$1,$2… $n | fields variables [$0 for entire line] |
NR | Number Row - keeps the current count of the row |
NF | Number field - keeps the current count if the fields |
FS | field separator [The default is “white space” ] |
RS | Record separator [The default is “New line character”] |
OFS | Specifies the Output separator. |
ORS | Specifies the Output separator. |
ARGC | Retrieves the number of passed parameters. |
ARGV | Retrieves the command line parameters. ARGV[1],ARGV[2]…. |
ENVIRON | Array of the shell environment variables and corresponding values. |
IGNORECASE | To ignore the character case. |
AWK Control Statements
- if (condition) statement [ else statement ]
- while (condition) statement
- do statement while (condition)
- for (expr1; expr2; expr3) statement
- for (var in array) statement
- break
- continue
Examples
Employee file employee.txt
which having employee records
1ajay manager account 45000
2sunil clerk account 25000
3varun manager sales 50000
4amit manager account 47000
5tarun peon sales 15000
6deepak clerk sales 23000
7sunil peon sales 13000
8satvik director purchase 80000
Print line by line from file
1awk '{print}' employee.txt
2
3#Output
4ajay manager account 45000
5sunil clerk account 25000
6varun manager sales 50000
7amit manager account 47000
8tarun peon sales 15000
9deepak clerk sales 23000
10sunil peon sales 13000
11satvik director purchase 80000
Print the lines which match the given pattern
1awk '/manager/ {print}' employee.txt
2
3# Output:
4ajay manager account 45000
5varun manager sales 50000
6amit manager account 47000
Splitting a Line Into Fields
1awk '{print $1,$4}' employee.txt
2
3#Output:
4ajay 45000
5sunil 25000
6varun 50000
7amit 47000
8tarun 15000
9deepak 23000
10sunil 13000
11satvik 80000
More Examples
1# To find/check for any string in any specific column
2awk '{ if($3 == "B6") print $0;}' geeksforgeeks.txt
3
4# To print the squares of first numbers from 1 to 6
5awk 'BEGIN { for(i=1;i<=6;i++) print "square of", i, "is",i*i; }'
6
7# Output
8square of 1 is 1
9square of 2 is 4
10square of 3 is 9
11square of 4 is 16
12square of 5 is 25
13square of 6 is 36
14
15# Use of NR built-in variables (Display Line Number)
16awk '{print NR,$0}' employee.txt
17
18# Output
191 ajay manager account 45000
202 sunil clerk account 25000
213 varun manager sales 50000
224 amit manager account 47000
235 tarun peon sales 15000
246 deepak clerk sales 23000
257 sunil peon sales 13000
268 satvik director purchase 80000
27
28# Use of NF built-in variables (Display Last Field)
29awk '{print $1,$NF}' employee.txt
30
31# Output
32ajay 45000
33sunil 25000
34varun 50000
35amit 47000
36tarun 15000
37deepak 23000
38sunil 13000
39satvik 80000
40
41
42# Another use of NR built-in variables (Display Line From 3 to 6)
43awk 'NR==3, NR==6 {print NR,$0}' employee.txt
44
45# Output
463 varun manager sales 50000
474 amit manager account 47000
485 tarun peon sales 15000
496 deepak clerk sales 23000
50
51# To count the lines in a file:
52awk 'END { print NR }' employee.txt
53
54# To find/check for any string in any specific column
55awk '{ if($3 == "B6") print $0;}' employee.txt
56
57# To print the squares of first numbers from 1 to n say 6
58awk 'BEGIN { for(i=1;i<=6;i++) print "square of", i, "is",i*i; }'
59
60# Output
61square of 1 is 1
62square of 2 is 4
63square of 3 is 9
64square of 4 is 16
65square of 5 is 25
66square of 6 is 36