Shell script - User management script
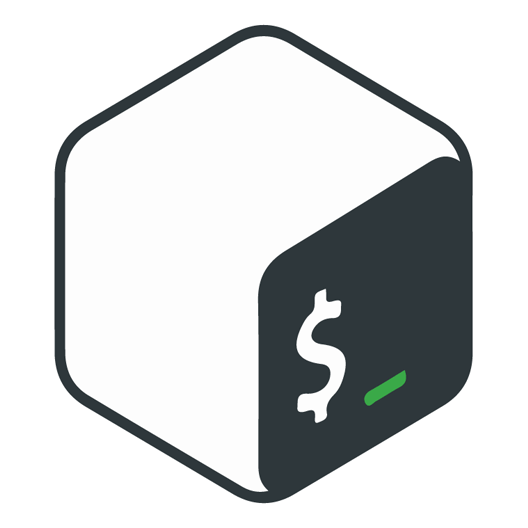
Below is an example of a shell script designed to manage users in Linux. This script includes various functionalities such as creating new users, listing existing users, resetting passwords, locking/unlocking users, backing up user data, deleting users, and more.
Feel free to review and utilize this script as needed.
Example
1#!/bin/bash
2
3# Envs
4# ---------------------------------------------------\
5PATH=$PATH:/bin:/sbin:/usr/bin:/usr/sbin:/usr/local/bin:/usr/local/sbin
6SCRIPT_PATH=$(cd `dirname "${BASH_SOURCE[0]}"` && pwd)
7cd $SCRIPT_PATH
8
9# Vars
10# ---------------------------------------------------\
11ME=`basename "$0"`
12BACKUPS=$SCRIPT_PATH/backups
13SERVER_NAME=`hostname`
14SERVER_IP=`hostname -I | cut -d' ' -f1`
15LOG=$SCRIPT_PATH/actions.log
16DISTRO_UNAME=`uname`
17
18# Output messages
19# ---------------------------------------------------\
20RED='\033[0;91m'
21GREEN='\033[0;92m'
22CYAN='\033[0;96m'
23YELLOW='\033[0;93m'
24PURPLE='\033[0;95m'
25BLUE='\033[0;94m'
26BOLD='\033[1m'
27WHiTE="\e[1;37m"
28NC='\033[0m'
29
30ON_SUCCESS="DONE"
31ON_FAIL="FAIL"
32ON_ERROR="Oops"
33ON_CHECK="✓"
34
35function _printPoweredBy()
36{
37 cat <<"EOF"
38 _ _ __ __
39 / \ _ __ __ _ _ __ __| | \ \ / / _ __ _ ___
40 / _ \ | '_ \ / _` | '_ \ / _` | \ \ / / | | |/ _` / __|
41 / ___ \| | | | (_| | | | | (_| | \ V /| |_| | (_| \__ \
42/_/ \_\_| |_|\__,_|_| |_|\__,_| \_/ \__, |\__,_|___/
43 |___/
44EOF
45}
46
47Info() {
48 echo -en "[${1}] ${GREEN}${2}${NC}\n"
49}
50
51Warn() {
52 echo -en "[${1}] ${PURPLE}${2}${NC}\n"
53}
54
55Success() {
56 echo -en "[${1}] ${GREEN}${2}${NC}\n"
57}
58
59Error () {
60 echo -en "[${1}] ${RED}${2}${NC}\n"
61}
62
63Splash() {
64 echo -en "${WHiTE} ${1}${NC}\n"
65}
66
67space() {
68 echo -e ""
69}
70
71
72# Functions
73# ---------------------------------------------------\
74
75logthis() {
76
77 echo "$(date): $(whoami) - $@" >> "$LOG"
78 # "$@" 2>> "$LOG"
79}
80
81isRoot() {
82 if [ $(id -u) -ne 0 ]; then
83 Error "You must be root user to continue"
84 exit 1
85 fi
86 RID=$(id -u root 2>/dev/null)
87 if [ $? -ne 0 ]; then
88 Error "User root no found. You should create it to continue"
89 exit 1
90 fi
91 if [ $RID -ne 0 ]; then
92 Error "User root UID not equals 0. User root must have UID 0"
93 exit 1
94 fi
95}
96
97# Checks supporting distros
98checkDistro() {
99 # Checking distro
100 if [ -e /etc/centos-release ]; then
101 DISTRO=`cat /etc/redhat-release | awk '{print $1,$4}'`
102 RPM=1
103 elif [ -e /etc/fedora-release ]; then
104 DISTRO=`cat /etc/fedora-release | awk '{print ($1,$3~/^[0-9]/?$3:$4)}'`
105 RPM=2
106 elif [ -e /etc/os-release ]; then
107 DISTRO=`lsb_release -d | awk -F"\t" '{print $2}'`
108 RPM=0
109 DEB=1
110 fi
111
112 if [[ "$DISTRO_UNAME" == 'Linux' ]]; then
113 _LINUX=1
114 Warn "Server info" "${SERVER_NAME} ${SERVER_IP} (${DISTRO}"
115 else
116 _LINUX=0
117 Error "Error" "Your distribution is not supported (yet)"
118 fi
119}
120
121# Yes / No confirmation
122confirm() {
123 # call with a prompt string or use a default
124 read -r -p "${1:-Are you sure? [y/N]} " response
125 case "$response" in
126 [yY][eE][sS]|[yY])
127 true
128 ;;
129 *)
130 false
131 ;;
132 esac
133}
134
135check_bkp_folder() {
136 if [[ ! -d "$BACKUPS" ]]; then
137 mkdir -p $BACKUPS
138 fi
139}
140
141gen_pass() {
142 local l=$1
143 [ "$l" == "" ] && l=9
144 tr -dc A-Za-z0-9 < /dev/urandom | head -c ${l} | xargs
145}
146
147create_user() {
148
149 space
150 read -p "Enter user name: " user
151
152 if id -u "$user" >/dev/null 2>&1; then
153 Error "Error" "User $user exists. Try to set another user name."
154 else
155 Info "Info" "User $user will be create.."
156
157 local pass=$(gen_pass)
158
159 if confirm "Promote user to admin? (y/n or enter for n)"; then
160 useradd -m -s /bin/bash -G wheel ${user}
161 echo "%$user ALL=(ALL) NOPASSWD: ALL" > /etc/sudoers.d/$user
162 else
163 useradd -m -s /bin/bash ${user}
164 fi
165
166 # set password
167 echo "$user:$pass" | chpasswd
168
169 Info "Info" "User created. Name: $user. Password: $pass"
170 logthis "User created. Name: $user. Password: $pass"
171
172 fi
173 space
174
175}
176
177list_users() {
178 space
179 Info "Info" "List of /bin/bash users: "
180 # grep 'bash' /etc/passwd | cut -d: -f1
181 users=$(awk -F: '$7=="/bin/bash" { print $1}' /etc/passwd)
182 for user in $users
183 do
184 echo "User: $user , $(id $user | cut -d " " -f 1)"
185 done
186 root_info=$(cat /etc/passwd | grep root)
187 Info "Root info" "${root_info}"
188 space
189}
190
191reset_password() {
192 space
193 while :
194 do
195 read -p "Enter user name: " user
196 if id $user &> /etc/null
197 then
198
199 if confirm "Generate password automatically? (y/n or enter for n)"; then
200 local pass=$(gen_pass)
201 echo "$user:$pass" | chpasswd
202 Info "Info" "Password changed. Name: $user. Password: $pass"
203 logthis "Password changed. Name: $user. Password: $pass"
204 else
205 read -p "Enter passwords: " password
206 echo "$password" | passwd --stdin $user
207 Info "Info" "Password changed. Name: $user. Password: $password"
208 logthis "Password changed. Name: $user. Password: $password"
209 fi
210 space
211 return 0
212 else
213 Error "Error" "User $user does not found!"
214 space
215 fi
216 done
217
218}
219
220lock_user() {
221
222 space
223 while :
224 do
225 read -p "Enter user name: " user
226 if [ -z $user ]
227 then
228 Error "Error" "Username can't be empty"
229 else
230 if id $user &> /etc/null
231 then
232 passwd -l $user
233 Info "Info" "User $user locked"
234 logthis "User $user locked"
235 space
236 return 0
237 else
238 Error "Error" "User $user does not found!"
239 space
240 fi
241 fi
242 done
243}
244
245unlock_user() {
246 space
247 while :
248 do
249 read -p "Enter user name: " user
250 if [ -z $user ]
251 then
252 Error "Error" "Username can't be empty"
253 else
254 if id $user &> /etc/null
255 then
256
257 local locked=$(cat /etc/shadow | grep $user | grep !)
258
259 if [[ -z $locked ]]; then
260 Info "Info" "User $user not locked"
261 else
262 passwd -u $user
263 Info "Info" "User $user unlocked"
264 logthis "User $user unlocked"
265 fi
266 space
267 return 0
268 else
269 Error "Error" "User $user does not found!"
270 space
271 fi
272 fi
273 done
274}
275
276list_locked_users() {
277 cat /etc/shadow | grep '!'
278}
279
280backup_user() {
281 space
282 while :
283 do
284 read -p "Enter user name: " user
285 if [ -z $user ]
286 then
287 Error "Error" "Username can't be empty"
288 else
289 if id $user &> /etc/null
290 then
291 check_bkp_folder
292 homedir=$(grep ${user}: /etc/passwd | cut -d ":" -f 6)
293 Info "Info" "Home directory for $user is $homedir "
294 Info "Info" "Creating..."
295 ts=$(date +%F)
296 tar -zcvf $BACKUPS/${user}-${ts}.tar.gz $homedir
297 Info "Info" "Backup for $user created with name ${user}-${ts}.tar.gz"
298 space
299 return 0
300 else
301 Error "Error" "User $user does not found!"
302 space
303 return 1
304 fi
305 fi
306 done
307}
308
309generate_ssh_key() {
310 space
311 while :
312 do
313 read -p "Enter user name: " user
314 if [ -z $user ]
315 then
316 Error "Error" "Username can't be empty"
317 else
318 if id $user &> /etc/null
319 then
320 local sshf="/home/$user/.ssh"
321 if [[ ! -d "$sshf" ]]; then
322 mkdir -p $sshf
323 chown $user:$user $sshf
324 chmod 700 $sshf
325 fi
326
327 su - $user -c "ssh-keygen -t rsa -b 4096 -C '${user}@local' -f ~/.ssh/id_rsa_${user} -N ''"
328 space
329 Info "Info" "User PUB key:"
330 space
331 su - $user -c "cat ~/.ssh/id_rsa_${user}.pub"
332 space
333 logthis "User $user ssh key is created - id_rsa_$user"
334 return 0
335 else
336 Error "Error" "User $user does not found!"
337 space
338 return 1
339 fi
340 fi
341 done
342}
343
344delete_user() {
345 space
346 while :
347 do
348 read -p "Enter user name: " user
349 if [ -z $user ]
350 then
351 Error "Error" "Username can't be empty"
352 else
353 if id $user &> /etc/null
354 then
355
356 if confirm "Completely delete user (y/n or press enter for n)"; then
357 userdel -r -f $user
358 if [[ -f /etc/sudoers.d/$user ]]; then
359 yes | rm -r /etc/sudoers.d/$user
360 fi
361
362 Info "Info" "User $user deleted"
363 space
364 fi
365 return 0
366 else
367 Error "Error" "User $user does not found!"
368 space
369 return 1
370 fi
371 fi
372 done
373}
374
375promote_user() {
376 space
377 while :
378 do
379 read -p "Enter user name: " user
380 if [ -z $user ]
381 then
382 Error "Error" "Username can't be empty"
383 else
384 if id $user &> /etc/null
385 then
386
387 if id $user | grep wheel &> /etc/null
388 then
389 Info "Info" "User already promoted to wheel group"
390 space
391 else
392 usermod -aG wheel $user
393 echo "%$user ALL=(ALL) NOPASSWD: ALL" > /etc/sudoers.d/$user
394 logthis "User $user promoted to wheel"
395 Info "Info" "User promoted to wheel group"
396 space
397 fi
398 return 0
399 else
400 Error "Error" "User $user does not found!"
401 space
402 return 1
403 fi
404 fi
405 done
406}
407
408degrate_user() {
409 space
410 while :
411 do
412 read -p "Enter user name: " user
413 if [ -z $user ]
414 then
415 Error "Error" "Username can't be empty"
416 else
417 if id $user &> /etc/null
418 then
419
420 if id $user | grep wheel &> /etc/null
421 then
422 Info "Info" "User already promoted to wheel group. Degrating..."
423 gpasswd -d $user wheel
424 yes | rm -r /etc/sudoers.d/$user
425 space
426 else
427 Info "Info" "User not promoted to wheel group"
428 space
429 fi
430 return 0
431 else
432 Error "Error" "User $user does not found!"
433 space
434 return 1
435 fi
436 fi
437 done
438}
439
440# Actions
441# ---------------------------------------------------\
442isRoot
443checkDistro
444_printPoweredBy
445# User menu rotator
446 while true
447 do
448 PS3='Please enter your choice: '
449 options=(
450 "Create new user"
451 "List users"
452 "Reset password for user"
453 "Lock user"
454 "Unlock user"
455 "List all locked users"
456 "Backup user"
457 "Generate SSH key for user"
458 "Promote user to admin"
459 "Degrate user from admin"
460 "Delete user"
461 "Quit"
462 )
463 select opt in "${options[@]}"
464 do
465 case $opt in
466 "Create new user")
467 create_user
468 break
469 ;;
470 "List users")
471 list_users
472 break
473 ;;
474 "Reset password for user")
475 reset_password
476 break
477 ;;
478 "Lock user")
479 lock_user
480 break
481 ;;
482 "Unlock user")
483 unlock_user
484 break
485 ;;
486 "List all locked users")
487 list_locked_users
488 break
489 ;;
490 "Backup user")
491 backup_user
492 break
493 ;;
494 "Generate SSH key for user")
495 generate_ssh_key
496 break
497 ;;
498 "Delete user")
499 delete_user
500 break
501 ;;
502 "Promote user to admin")
503 promote_user
504 break
505 ;;
506 "Degrate user from admin")
507 degrate_user
508 break
509 ;;
510 "Quit")
511 Info "Exit" "Bye"
512 exit
513 ;;
514 *) echo invalid option;;
515 esac
516 done
517 done