Kubernetes - Cheat Sheet
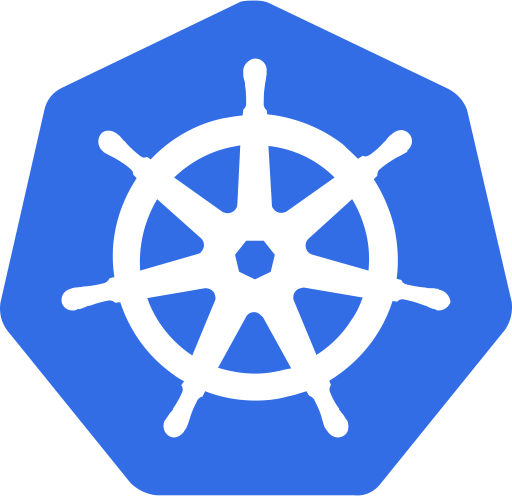
Cluster Info
- Get clusters
1kubectl config get-clusters
- Get cluster info.
1kubectl cluster-info
2Kubernetes master is running at https://172.17.0.58:8443
Contexts
A context is a cluster, namespace and user.
- Get a list of contexts.
1kubectl config get-contexts
- Get the current context.
1kubectl config current-context
- Switch current context.
1kubectl config use-context docker-desktop
- Set default namesapce
1kubectl config set-context $(kubectl config current-context) --namespace=my-namespace
Get Commands
1kubectl get all
2kubectl get namespaces
3kubectl get configmaps
4kubectl get nodes
5kubectl get pods
6kubectl get rs
7kubectl get svc kuard
8kubectl get endpoints kuard
Additional switches that can be added to the above commands:
-o wide
- Show more information.--watch
or-w
- watch for changes.
Namespaces
--namespace
- Get a resource for a specific namespace.
You can set the default namespace for the current context like so:
1kubectl config set-context $(kubectl config current-context) --namespace=my-namespace
Labels
- Get pods showing labels.
1kubectl get pods --show-labels
- Get pods by label.
1kubectl get pods -l environment=production,tier!=frontend
2kubectl get pods -l 'environment in (production,test),tier notin (frontend,backend)'
Describe Command
1kubectl describe nodes [id]
2kubectl describe pods [id]
3kubectl describe rs [id]
4kubectl describe svc kuard [id]
5kubectl describe endpoints kuard [id]
Delete Command
1kubectl delete nodes [id]
2kubectl delete pods [id]
3kubectl delete rs [id]
4kubectl delete svc kuard [id]
5kubectl delete endpoints kuard [id]
Force a deletion of a pod without waiting for it to gracefully shut down
1kubectl delete pod-name --grace-period=0 --force
Create vs Apply
kubectl create
can be used to create new resources while kubectl apply
inserts or updates resources while maintaining any manual changes made like scaling pods.
--record
- Add the current command as an annotation to the resource.--recursive
- Recursively look for yaml in the specified directory.
Create Pod
1kubectl run kuard --generator=run-pod/v1 --image=gcr.io/kuar-demo/kuard-amd64:1 --output yaml --export --dry-run > kuard-pod.yml
2kubectl apply -f kuard-pod.yml
Create Deployment
1kubectl run kuard --image=gcr.io/kuar-demo/kuard-amd64:1 --output yaml --export --dry-run > kuard-deployment.yml
2kubectl apply -f kuard-deployment.yml
Create Service
1kubectl expose deployment kuard --port 8080 --target-port=8080 --output yaml --export --dry-run > kuard-service.yml
2kubectl apply -f kuard-service.yml
Export YAML for New Pod
1kubectl run my-cool-app —-image=me/my-cool-app:v1 --output yaml --export --dry-run > my-cool-app.yaml
Export YAML for Existing Object
1kubectl get deployment my-cool-app --output yaml --export > my-cool-app.yaml
Logs
1kubectl logs -l app=kuard
2
3# Get logs for previously terminated container.
4kubectl logs POD_NAME --previous
5
6# Watch logs in real time.
7kubectl attach POD_NAME
8
9# Copy log files out of pod (Requires `tar` binary in container).
10kubectl cp POD_NAME:/var/log .
Port Forward
1kubectl port-forward deployment/kuard 8080:8080
Scaling
- Update replicas.
1kubectl scale deployment nginx-deployment --replicas=10
Autoscaling
- Set autoscaling config.
1kubectl autoscale deployment nginx-deployment --min=10 --max=15 --cpu-percent=80
Rollout
1# Get rollout status.
2kubectl rollout status deployment/nginx-deployment
3
4# Get rollout history.
5
6kubectl rollout history deployment/nginx-deployment
7kubectl rollout history deployment/nginx-deployment --revision=2
8
9# Undo a rollout.
10
11kubectl rollout undo deployment/nginx-deployment
12kubectl rollout undo deployment/nginx-deployment --to-revision=2
13
14# Pause/resume a rollout
15
16kubectl rollout pause deployment/nginx-deployment
17kubectl rollout resume deploy/nginx-deployment
Examples of get commands
1# Get commands with basic output
2kubectl get services # List all services in the namespace
3kubectl get pods --all-namespaces # List all pods in all namespaces
4kubectl get pods -o wide # List all pods in the namespace, with more details
5kubectl get deployment my-dep # List a particular deployment
6kubectl get pods --include-uninitialized # List all pods in the namespace, including uninitialized ones
7
8# Describe commands with verbose output
9kubectl describe nodes my-node
10kubectl describe pods my-pod
11
12kubectl get services --sort-by=.metadata.name # List Services Sorted by Name
13
14# List pods Sorted by Restart Count
15kubectl get pods --sort-by='.status.containerStatuses[0].restartCount'
16
17# Get the version label of all pods with label app=cassandra
18kubectl get pods --selector=app=cassandra rc -o \
19 jsonpath='{.items[*].metadata.labels.version}'
20
21# Get all worker nodes (use a selector to exclude results that have a label
22# named 'node-role.kubernetes.io/master')
23kubectl get node --selector='!node-role.kubernetes.io/master'
24
25# Get all running pods in the namespace
26kubectl get pods --field-selector=status.phase=Running
27
28# Get ExternalIPs of all nodes
29kubectl get nodes -o jsonpath='{.items[*].status.addresses[?(@.type=="ExternalIP")].address}'
30
31# List Names of Pods that belong to Particular RC
32# "jq" command useful for transformations that are too complex for jsonpath, it can be found at https://stedolan.github.io/jq/
33sel=${$(kubectl get rc my-rc --output=json | jq -j '.spec.selector | to_entries | .[] | "\(.key)=\(.value),"')%?}
34echo $(kubectl get pods --selector=$sel --output=jsonpath={.items..metadata.name})
35
36# Show labels for all pods (or any other Kubernetes object that supports labelling)
37# Also uses "jq"
38for item in $( kubectl get pod --output=name); do printf "Labels for %s\n" "$item" | grep --color -E '[^/]+$' && kubectl get "$item" --output=json | jq -r -S '.metadata.labels | to_entries | .[] | " \(.key)=\(.value)"' 2>/dev/null; printf "\n"; done
39
40# Check which nodes are ready
41JSONPATH='{range .items[*]}{@.metadata.name}:{range @.status.conditions[*]}{@.type}={@.status};{end}{end}' \
42 && kubectl get nodes -o jsonpath="$JSONPATH" | grep "Ready=True"
43
44# List all Secrets currently in use by a pod
45kubectl get pods -o json | jq '.items[].spec.containers[].env[]?.valueFrom.secretKeyRef.name' | grep -v null | sort | uniq
46
47# List Events sorted by timestamp
48kubectl get events --sort-by=.metadata.creationTimestamp