Yaml (Yet Another Markup Language)
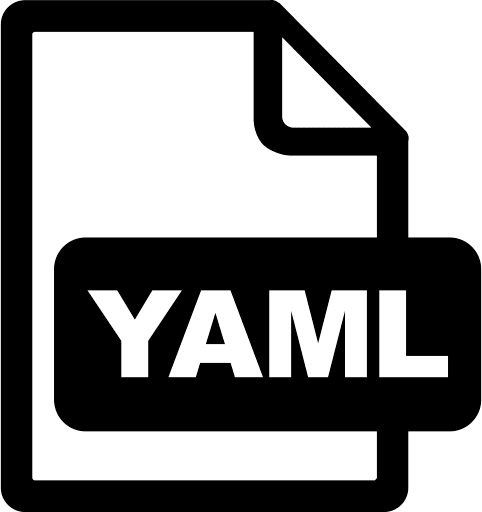
YAML (“Yet Another Markup Language”) is a human-readable data serialization language. It is commonly used for configuration files
Basic Rules
YAML is case sensitive.
YAML does not allow the use of tabs. Spaces are used instead as tabs because tabs are not universally supported.
Scalar types (Datatypes)
1n1: 1 # integer
2n2: 1.234 # float
3
4s1: 'abc' # string
5s2: "abc" # string
6s3: abc # string
7
8b: false # boolean type
9
10d: 2015-04-05 # date type
11
12e: !!str 123 # a string, disambiguated by explicit type
13f: !!str Yes # a string via explicit type
14g: !!float 123 # also a float via explicit data type prefixed by (!!)
15
Equavalent JSON
1{
2 "n1": 1,
3 "n2": 1.234,
4 "s1": "abc",
5 "s2": "abc",
6 "s3": "abc",
7 "b": false,
8 "d": "2015-04-05"
9}
Variables Reference
1some_thing: &VAR_NAME foobar
2other_thing: *VAR_NAME
Equavalent JSON
1{
2 "some_thing": "foobar",
3 "other_thing": "foobar"
4}
Sequence
1object:
2 attributes:
3 - a1
4 - a2
5 - a3
6 methods: [getter, setter]
Equavalent JSON
1{
2 "object": {
3 "attributes": ["a1", "a2", "a3"],
4 "methods": ["getter", "setter"]
5 }
6}
Sequence of sequences
1my_sequences:
2 - [1, 2, 3]
3 - [4, 5, 6]
Equavalent JSON
1{
2 "my_sequences": [
3 [1, 2, 3],
4 [4, 5, 6]
5 ]
6}
Comments
1# A single line comment example
2
3# block level comment example
4# comment line 1
5# comment line 2
6# comment line 3
Multiline strings
1description: |
2 hello
3 world
Equavalent JSON
1{"description": "hello\nworld\n"}
Folded text
1description: >
2 hello
3 world
Equavalent JSON
1{"description": "hello world\n"}
Hashes
1jack:
2 id: 1
3 name: Franc
4 salary: 5000
5 hobby:
6 - a
7 - b
8 loc: {country: "A", city: "A-A"}
Equavalent JSON
1{
2 "jack": {
3 "id": 1,
4 "name": "Franc",
5 "salary": 5000,
6 "hobby": ["a", "b"],
7 "loc": {
8 "country": "A", "city": "A-A"
9 }
10 }
11}
Nested dictionaries
1Employee:
2 id: 1
3 name: "Franc"
4 department:
5 name: "Sales"
6 depid: "11"
Equavalent JSON
1{
2 "Employee": {
3 "id": 1,
4 "name": "Franc",
5 "department": {
6 "name": "Sales",
7 "depid": "11"
8 }
9 }
10}
Sequence of dictionaries
1children:
2 - name: Jimmy Smith
3 age: 15
4 - name: Jenny Smith
5 age: 12
Equavalent JSON
1{
2 "children": [
3 {"name": "Jimmy Smith", "age": 15},
4 {"name": "Jenny Smith", "age": 12}
5 ]
6}
Set
1set1: !!set
2 ? one
3 ? two
4set2: !!set {'one', "two"}
Equavalent JSON
1{
2 "set1": {"one": null, "two": null},
3 "set2": {"one": null, "two": null}
4}
Inheritance
1parent: &defaults
2 a: 2
3 b: 3
4
5child:
6 <<: *defaults
7 b: 4
Equavalent JSON
1{
2"parent": {"a": 2, "b": 3},
3"child": {"a": 2, "b": 4}
4}
Reference
1values: &ref
2 - These values
3 - will be reused below
4
5other_values:
6 i_am_ref: *ref
Equavalent JSON
1{
2 "values": [
3 "These values",
4 "will be reused below"
5 ],
6 "other_values": {
7 "i_am_ref": [
8 "These values",
9 "will be reused below"
10 ]
11 }
12}
Documents
1---
2document: this is doc 1
3---
4document: this is doc 2
5...