KISS principle
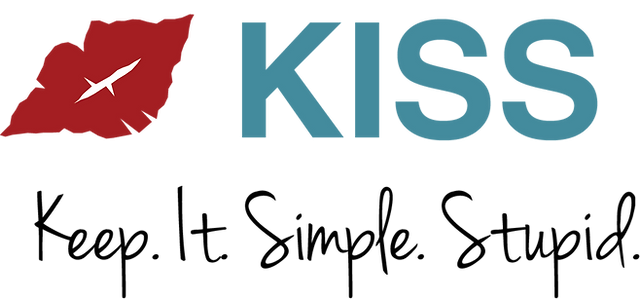
The KISS Principle
, which stands for Keep It Simple, Stupid
is a design and software development principle that advocates for simplicity. The main idea behind KISS is that systems and code should be kept as simple as possible to avoid unnecessary complexity. By doing so, the system becomes easier to understand, maintain, and modify, leading to more reliable and effective solutions.
Key Concepts of the KISS Principle
Simplicity
: The core of the KISS principle is to avoid over-complicating things. Whether designing a system, writing code, or creating user interfaces, simplicity should be a priority. This means avoiding unnecessary features, overly complex algorithms, or convoluted logic.Readability
: Simple code is easier to read and understand. When code is straightforward, developers can quickly grasp its functionality, which reduces the time spent deciphering logic and makes onboarding new team members easier.Maintainability
: Simplicity leads to maintainable code. Code that is easy to understand is also easier to maintain and modify. When changes are needed, developers can confidently make updates without fear of breaking hidden dependencies or encountering unforeseen complexities.Avoiding Over-Engineering
: The KISS principle warns against trying to solve problems that do not yet exist or adding features that are not necessary. Over-engineering can lead to bloated systems that are harder to manage and prone to more bugs.
Examples of the KISS Principle in Practice
Without KISS: A function that tries to handle multiple tasks and ends up being complex and difficult to understand.
1def process_user_data(user):
2 if isinstance(user, dict):
3 if "name" in user and "email" in user:
4 if "@" in user["email"]:
5 # Process user data
6 print(f"Processing {user['name']} with email {user['email']}")
7 else:
8 raise ValueError("Invalid email format")
9 else:
10 raise KeyError("Missing required user information")
11 else:
12 raise TypeError("Invalid user type")
With KISS: Break down the function into smaller, more focused functions that handle specific tasks, making it easier to understand and maintain.
1def validate_user(user):
2 if not isinstance(user, dict):
3 raise TypeError("Invalid user type")
4 if "name" not in user or "email" not in user:
5 raise KeyError("Missing required user information")
6 if "@" not in user["email"]:
7 raise ValueError("Invalid email format")
8
9def process_user_data(user):
10 validate_user(user)
11 print(f"Processing {user['name']} with email {user['email']}")
Benefits of the KISS Principle
Easier Debugging and Testing
: Simpler code is easier to test and debug. When code is straightforward, it is easier to identify and fix issues, leading to fewer bugs and higher quality software.Faster Development
: By focusing on simplicity, development time can be reduced. Developers spend less time writing, reading, and understanding complex code, leading to faster iterations and delivery.Better Collaboration
: Simple code is easier for team members to understand, making collaboration more effective. Teams can work together more efficiently when everyone can quickly understand and contribute to the codebase.Scalability
: Simple solutions are often easier to scale. By avoiding unnecessary complexity, systems can grow and evolve more easily, adapting to new requirements and technologies.Lower Cost of Ownership
: Overly complex systems can be costly to maintain and update. By adhering to the KISS principle, organizations can reduce maintenance costs and extend the lifespan of their software.
Common Misconceptions about KISS
Simple Doesn’t Mean Lack of Functionality
: The KISS principle is not about removing essential features or dumbing down systems. It’s about avoiding unnecessary complexity and focusing on what truly matters.Simplicity Doesn’t Mean Inefficiency
: Simple solutions can be both effective and efficient. Simplicity does not imply cutting corners or ignoring performance. It means solving problems with the least amount of complexity.KISS Is Not Just for Code
: While often applied to coding, the KISS principle applies to all aspects of software development, including architecture, design, documentation, and process management.